Paying with Processing (Part Two)
Last time I ran an example sketch and then wrote my own that prints “Hello World” in bright colours. Now I want to play around with some of the basic shapes that Processing provides. From the documentation it looks like the 2D primitives are the arc, ellipse, line, point, quad, rect, and triangle.
The first one I’m going to play with is the rect() primitive, so I wrote a simple sketch to draw one. All it does is set the window size then draw a rectangle somewhere in the window.
void setup() { size(640, 480); smooth(); rect(30, 30, 155, 155); }
The rect() function takes four parameters as inputs, the first two are the x and y co-ordinates of where to start drawing. The second two are the height and width of the rectangle. Then I thought it would be cool if I made it follow the mouse, this as it turns out is really easy. In the draw function you get a couple of variables you can use for this, mouseX and mouseY. They hold the x and y co-ordinates of where the mouse is so all I have to do is put the call to rect() into the draw loop then use the mouse co-ordinates to set that starting position of the rectangle.
rect(mouseX, mouseY, 155, 155);
This code worked ok but the viewport filled up with loads of rectangles as you moved the mouse really quickly. I wanted just a single rectangle that followed the mouse. So I added some extra lines of code that drew another rectangle over the whole viewport to clear it then draw the smaller rectangle. I also thought it would look much nicer if the mouse cursor was always located in the centre of the rectangle rather than at the top left-hand corner. So to do this I subtracted half the width of the rectangle from the x co-ordinate or the mouse cursor, and did the same with the height and y co-ordinate. This kept the mouse cursor roughly in the middle of the rectangle.
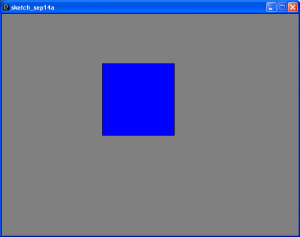
To draw the rectangle over the entire viewport I two other special variables, width and height. These are set to the width and height of the window you are using. The code for all of this is below.
void setup() { size(640, 480); smooth(); } void draw() { fill(128,128,128); rect(0, 0, width, height); fill(0, 0, 255); rect(mouseX-(155/2), mouseY-(155/2), 155, 155); }
Well that’s all for now. See ya!
graphics processing