An Analogue Clock in Processing
I came across some code I wrote in Processing a while ago so I thought Iβd post bits of it here. I made a really simple analogue clock. Below is s screenshot of what I came up with.
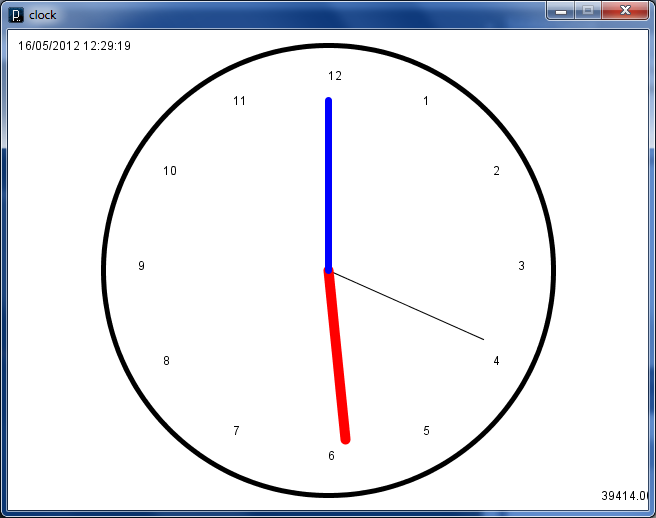
I wrote a small function that handles drawing a hand of the clock. This function is called for each hand of the code by the draw loop.
void draw_hand(long count, long modulo, long len) { float nx, ny; a = (2*PI/modulo) * count - (2*PI/4); nx = (width/2) + (Clock_Size/2-len) * cos(a); ny = (height/2) + (Clock_Size/2-len) * sin(a); strokeWeight(7); line(width/2, height/2, nx, ny); }
Then all we need to do in the draw loop is call the function for each of the hands of the clock. Here is an example of drawing the hours hand.
To put the ticks around the outside of the clock I used the code below.
fill(0,0,0); for( int i = 0; i < 12; i ++ ) { float angle = radians(i*30) - (2*PI/4); nx = (width/2) + (Clock_Size/2-35) * cos(angle); ny = (height/2) + (Clock_Size/2-35) * sin(angle); text(i == 0 ? 12 : i, nx, ny); }
Processing.js Version
I have got this code working fine using Processing.js. See it here
graphics processing programming